How To Convert A txt File To A PDF Using Python & FPDF
Part 1 of the flask feature series
TECHNICAL LEARNINGCODING
9/18/20233 min read
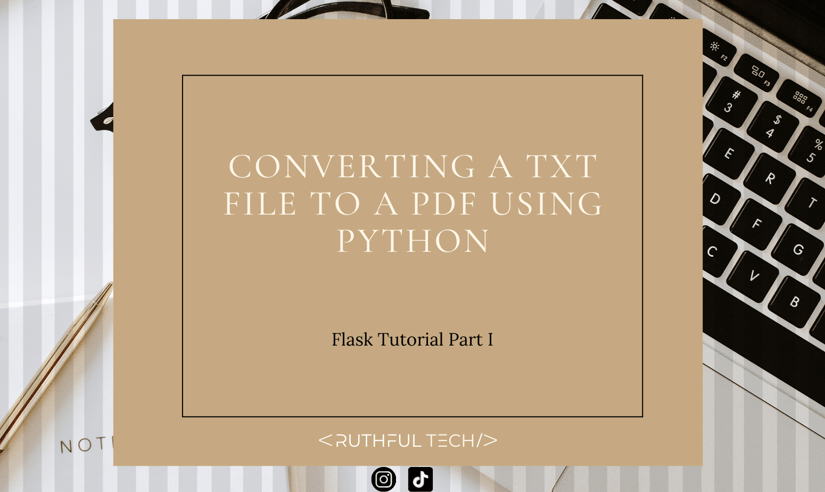
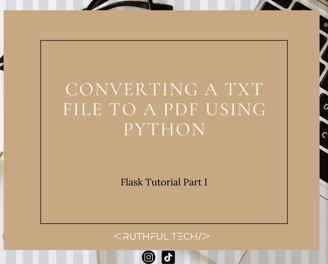
One of the reasons why I created a blog is to ‘learn in public’. This a big thing in the dev community (and quite a new concept for me coming from a background in Pharmacy). But I love the ability to share raw, uncut things and get feedback/ bond with other learners facing the same difficulties or triumphs. I have been working on a personal Flask app project.
One of the features I wanted to implement involved:
👩🏾💻Creating a txt file from specific data retrieved from an API based on inputs from the user
👩🏾💻Converting the txt file into a pdf file
👩🏾💻Allowing the user to download this pdf file onto their local device if desired
In this first post, we will look at how to convert a text file to a pdf using Python.
My text file is located in my project folder along with the Python script we'll be using to convert it. Here is it!:
We then create an instance of the FPDF class and assign it to a variable 'pdf'.
After, we call the .add_page() method to create a new page.
When a new page is created, we have to define the font and font size using the set_font() method.
The set_font() function takes in 3 arguments, the font name, style (if we want it bold (B), in italics (I) etc) and the font size. For this project, I only needed to specify the font and font size.
We open our pre-existing txt file in the ‘read’ mode.
Then we will iterate through each word in this file.
Next, we must print to our cells within our new pdf object. The cell method takes in many arguments, the ones we’ll be using are: width, height, the string to be printed, ln (which indicates where the current position should go after the call), and alignment.
NB: I've wrapped this in a try-except block to catch any errors as we are working with files!!
Finally, we call the output() method on our pdf object. This method takes in two arguments, the new file name of our converted pdf (must have a '.pdf' suffix), and our desired destination. Read more about it here.
For the purpose of this tutorial, I haven't specified a destination and by default, the pdf file will be placed in my project directory.
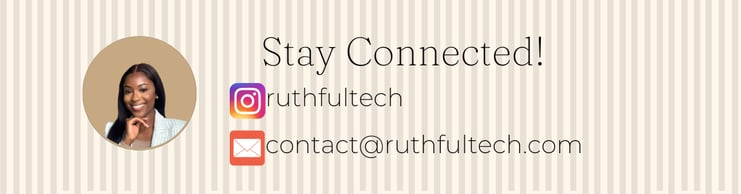
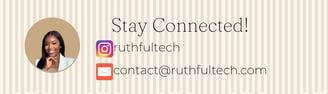
That's all for this tutorial.
Feel free to message me here with any questions or any other ways you've found for converting a txt file to a pdf.
If you want to learn more about fpdf and what else you can do with it, here is a great resource.
In the next post from this episode, we will be jumping over to the user interface to see how we can enable the user to download the pdf onto their desktop.
Credits to Carbon for their amazing code snippets feature!
Happy Coding!
Ruth
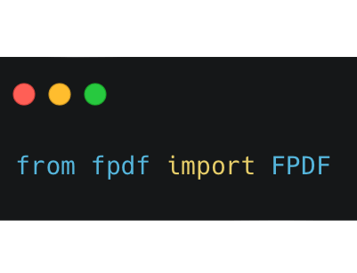
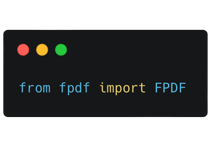
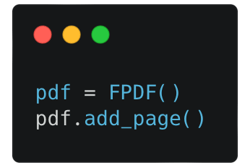
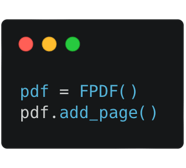
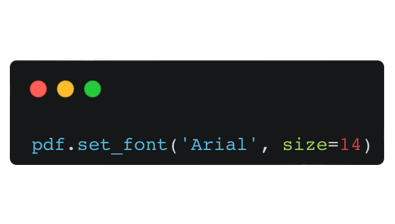
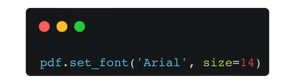
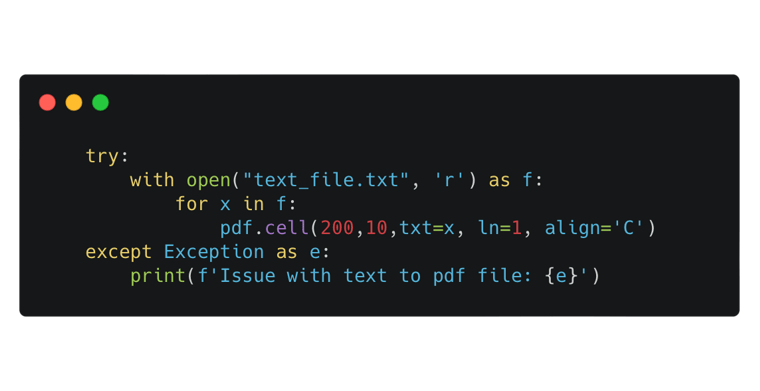
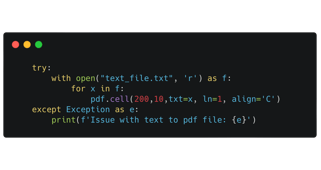
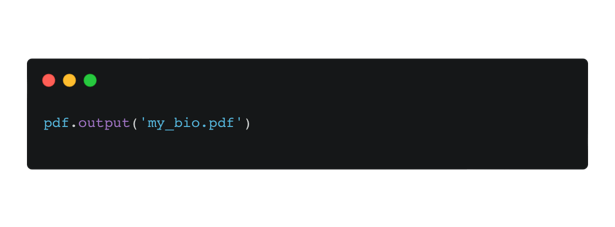
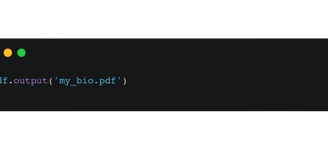
For the sake of reusability, I have placed the code in a function called 'convert_to_pdf' which takes in two arguments, a text file and the new file name. So altogether it is:
Next, we import the fpdf library into our python script
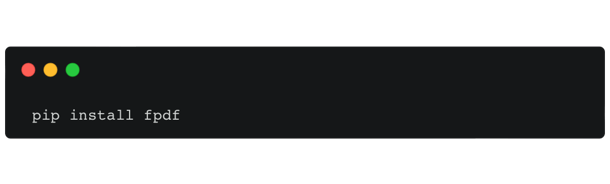
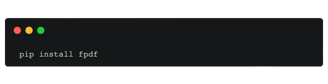
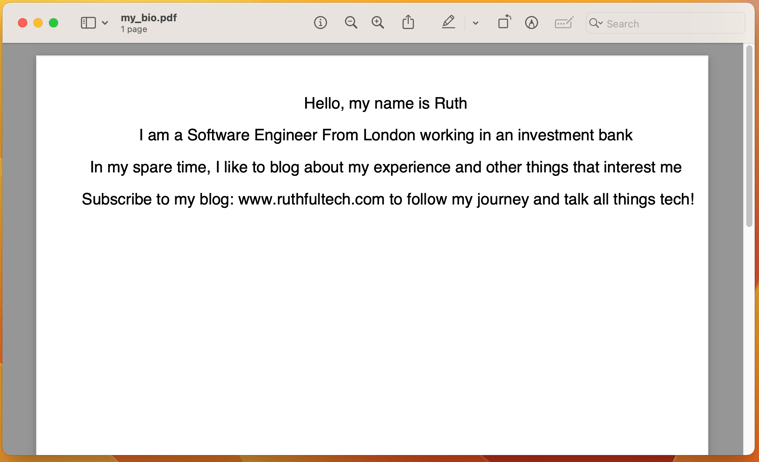
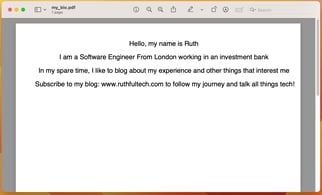
And here is my converted pdf! 🥳
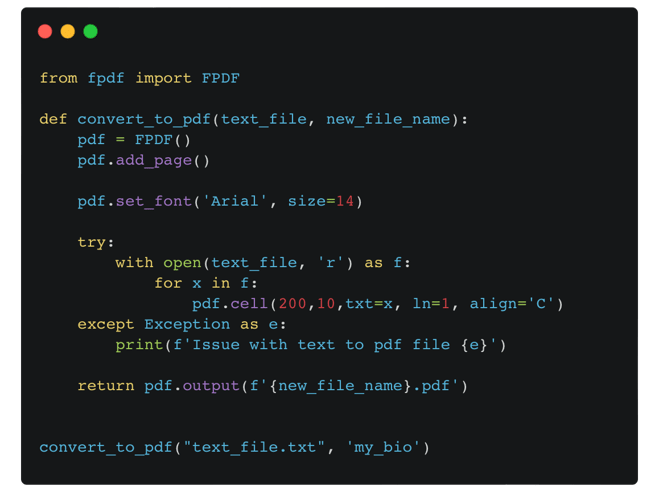
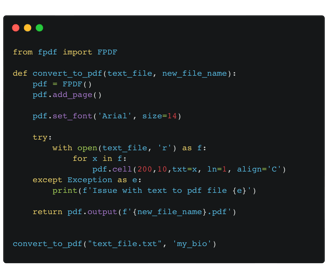
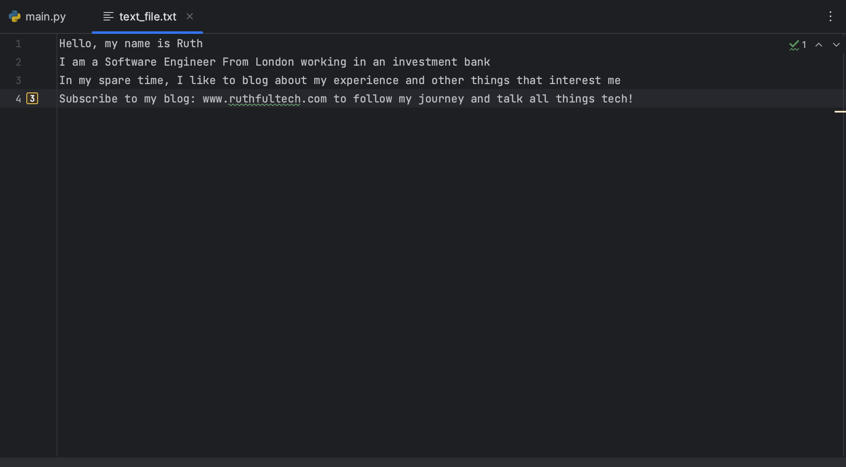
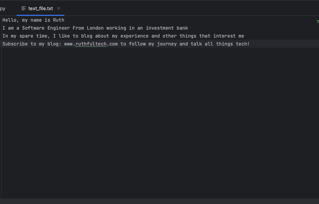
First, we install the fpdf library in the terminal using pip.